27
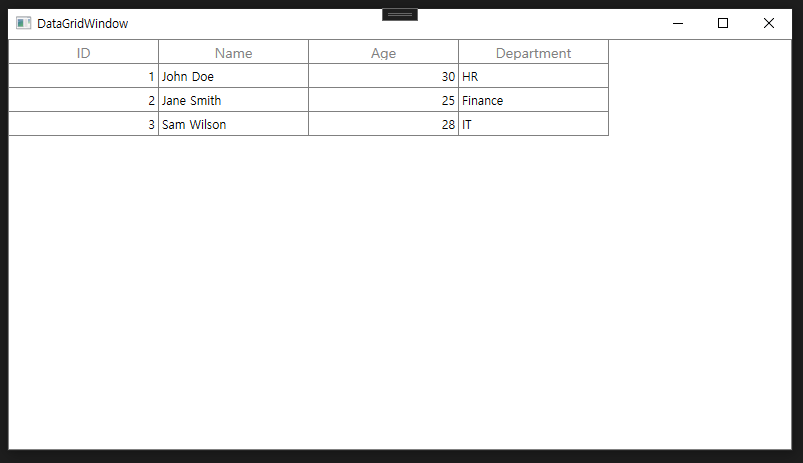
Syncfusion의 WPF GridControl을 사용하는 방법은 매우 직관적이며, 기본적으로 NuGet 패키지에서 Syncfusion WPF Library를 설치한 후 사용할 수 있습니다. 여기에서는 SfDataGrid
를 활용한 간단한 데이터 바인딩 예제를 보여드리겠습니다.
1. NuGet 패키지 설치
Syncfusion WPF 라이브러리를 설치합니다
Install-Package Syncfusion.SfGrid.WPF
2. XAML 설정
SfDataGrid
컨트롤을 XAML에 추가하고, 데이터를 바인딩합니다.
<Window x:Class="SyncfusionGridExample.MainWindow"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:d="http://schemas.microsoft.com/expression/blend/2008"
xmlns:mc="http://schemas.openxmlformats.org/markup-compatibility/2006"
xmlns:syncfusion="http://schemas.syncfusion.com/wpf"
mc:Ignorable="d"
Title="DataGridWindow" Height="450" Width="800">
<Grid>
<syncfusion:SfDataGrid x:Name="dataGrid"
AutoGenerateColumns="True"
ItemsSource="{Binding Employees}" />
</Grid>
</Window>
3. ViewModel 생성
MVVM 패턴을 따르는 예제에서 ViewModel을 정의합니다.
using System.Collections.ObjectModel;
namespace SyncfusionGridExample
{
public class MainViewModel
{
public ObservableCollection<Employee> Employees { get; set; }
public MainViewModel()
{
Employees = new ObservableCollection<Employee>
{
new Employee { ID = 1, Name = "John Doe", Age = 30, Department = "HR" },
new Employee { ID = 2, Name = "Jane Smith", Age = 25, Department = "Finance" },
new Employee { ID = 3, Name = "Sam Wilson", Age = 28, Department = "IT" }
};
}
}
public class Employee
{
public int ID { get; set; }
public string Name { get; set; }
public int Age { get; set; }
public string Department { get; set; }
}
}
4. Code-Behind에서 ViewModel 연결
MainWindow.xaml.cs
에서 DataContext를 ViewModel로 설정합니다.
using System.Windows;
namespace SyncfusionGridExample
{
public partial class MainWindow : Window
{
public MainWindow()
{
InitializeComponent();
DataContext = new MainViewModel();
}
}
}
5. 결과 사진
위 코드를 실행하면 다음과 같은 Syncfusion SfDataGrid
가 표시됩니다
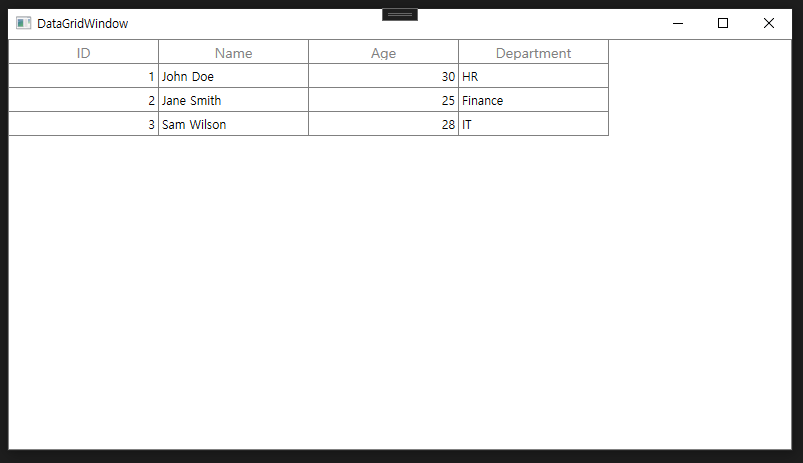
주요 옵션 및 기능
- 열 자동 생성
AutoGenerateColumns="True"
속성을 사용하면 데이터 모델에 기반하여 자동으로 열이 생성됩니다.
- 열 사용자 지정
- 열을 직접 정의하려면
GridTextColumn
을 사용합니다
- 열을 직접 정의하려면
<syncfusion:SfDataGrid.AutoGenerateColumns>
<syncfusion:GridTextColumn HeaderText="Employee ID" MappingName="ID" />
<syncfusion:GridTextColumn HeaderText="Full Name" MappingName="Name" />
<syncfusion:GridTextColumn HeaderText="Age" MappingName="Age" />
<syncfusion:GridTextColumn HeaderText="Dept." MappingName="Department" />
</syncfusion:SfDataGrid.AutoGenerateColumns>
- 정렬 및 필터링
AllowSorting="True"
및AllowFiltering="True"
속성을 사용하여 정렬 및 필터링 기능을 활성화할 수 있습니다.
- 행 편집
AllowEditing="True"
속성을 추가하면 사용자가 데이터를 직접 수정할 수 있습니다.