26
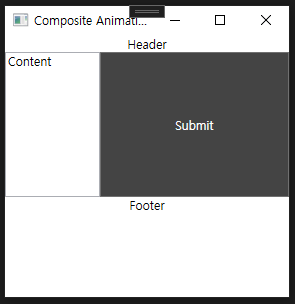
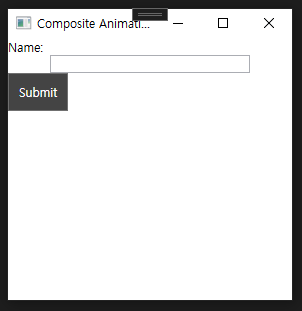
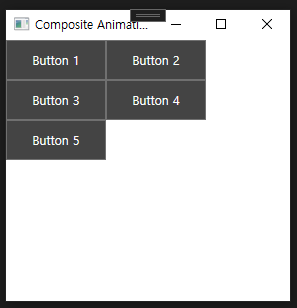
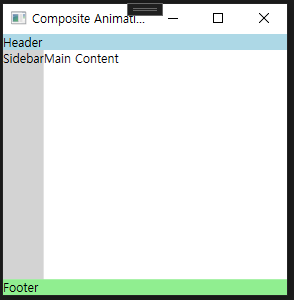

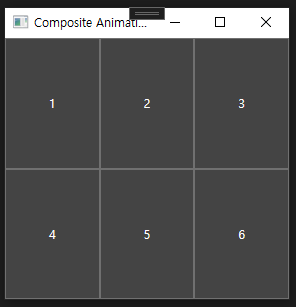
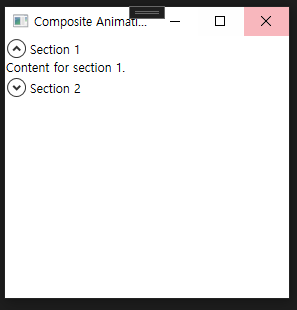
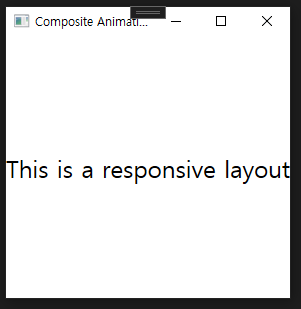
WPF의 레이아웃 시스템은 요소들을 배치하고 크기를 동적으로 조정하는 강력한 기능을 제공합니다. 주요 레이아웃 컨테이너로는 Grid, StackPanel, WrapPanel, DockPanel, Canvas 등이 있으며, 각각 특정 상황에 적합합니다.
아래에서는 각 레이아웃 컨테이너와 관련된 예제 코드와 설명을 제공합니다.
1. Grid
Grid는 행과 열로 요소를 정렬하는 가장 강력한 레이아웃 컨테이너입니다.RowDefinitions
와 ColumnDefinitions
를 통해 레이아웃을 정의하며, 상대 크기(*
), 고정 크기, 자동 크기(Auto
)를 지원합니다.
예제 코드
<Grid>
<Grid.RowDefinitions>
<RowDefinition Height="Auto" />
<RowDefinition Height="*" />
<RowDefinition Height="100" />
</Grid.RowDefinitions>
<Grid.ColumnDefinitions>
<ColumnDefinition Width="*" />
<ColumnDefinition Width="2*" />
</Grid.ColumnDefinitions>
<TextBlock Text="Header" Grid.Row="0" Grid.ColumnSpan="2" HorizontalAlignment="Center" />
<TextBox Text="Content" Grid.Row="1" Grid.Column="0" />
<Button Content="Submit" Grid.Row="1" Grid.Column="1" />
<TextBlock Text="Footer" Grid.Row="2" Grid.ColumnSpan="2" HorizontalAlignment="Center" />
</Grid>
실행사진
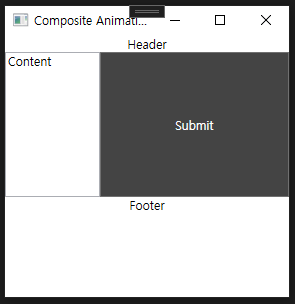
설명
- 행/열 크기:
Auto
: 자식 요소의 크기에 맞춰 크기 설정.*
: 사용 가능한 공간을 상대적으로 할당.- 고정값(예:
100
): 픽셀 크기 지정.
- Grid.Row, Grid.Column 속성을 통해 요소 배치.
2. StackPanel
StackPanel은 요소들을 수직 또는 수평으로 나란히 정렬합니다.
예제 코드
<StackPanel Orientation="Vertical">
<TextBlock Text="Name:" />
<TextBox Width="200" />
<Button Content="Submit" HorizontalAlignment="Left" />
</StackPanel>
실행사진
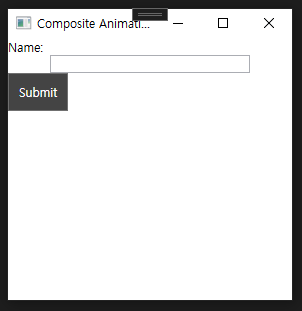
설명
- Orientation:
Vertical
: 수직 정렬 (기본값).Horizontal
: 수평 정렬.
- 자식 요소들은 순서대로 정렬되며 공간이 넘치면 잘립니다.
3. WrapPanel
WrapPanel은 공간이 부족할 경우 요소를 다음 줄로 이동하며 배치합니다.
예제 코드
<WrapPanel ItemWidth="100" ItemHeight="40">
<Button Content="Button 1" />
<Button Content="Button 2" />
<Button Content="Button 3" />
<Button Content="Button 4" />
<Button Content="Button 5" />
</WrapPanel>
실행사진
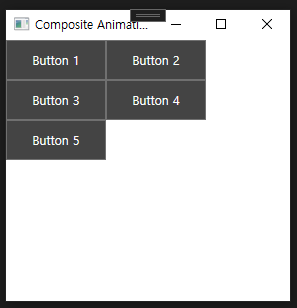
설명
- ItemWidth/ItemHeight: 자식 요소의 기본 크기 지정.
- 공간을 유연하게 활용할 수 있어 반응형 레이아웃에 유용.
4. DockPanel
DockPanel은 요소를 지정된 가장자리에 도킹합니다.
예제 코드
<DockPanel>
<TextBlock Text="Header" DockPanel.Dock="Top" Background="LightBlue" />
<TextBlock Text="Footer" DockPanel.Dock="Bottom" Background="LightGreen" />
<TextBlock Text="Sidebar" DockPanel.Dock="Left" Background="LightGray" />
<TextBlock Text="Main Content" Background="White" />
</DockPanel>
실행사진
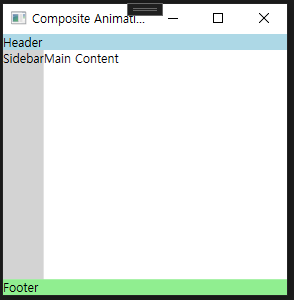
설명
- DockPanel.Dock: 요소를
Top
,Bottom
,Left
,Right
로 배치. - 마지막 요소는 기본적으로 남은 공간을 채웁니다.
5. Canvas
Canvas는 자식 요소를 절대 위치로 배치합니다.
예제 코드
<Canvas>
<Button Content="Button 1" Canvas.Left="50" Canvas.Top="20" />
<Button Content="Button 2" Canvas.Left="150" Canvas.Top="50" />
</Canvas>
실행사진

설명
- Canvas.Left, Canvas.Top: 절대 좌표 지정.
- 주로 고정된 위치가 필요한 요소에 적합.
6. UniformGrid
UniformGrid는 모든 셀에 동일한 크기를 부여하며, 자식 요소를 균등하게 배치합니다.
예제 코드
<UniformGrid Rows="2" Columns="3">
<Button Content="1" />
<Button Content="2" />
<Button Content="3" />
<Button Content="4" />
<Button Content="5" />
<Button Content="6" />
</UniformGrid>
실행사진
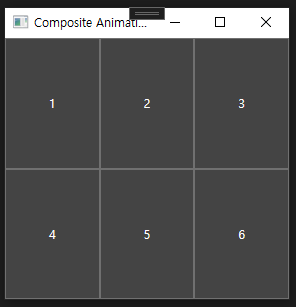
설명
- Rows/Columns: 행과 열의 개수 지정.
- 모든 셀의 크기가 동일.
7. Expander + ScrollViewer
레이아웃 요소와 ScrollViewer를 결합하여 스크롤 가능한 콘텐츠를 추가할 수 있습니다.
예제 코드
<ScrollViewer VerticalScrollBarVisibility="Auto">
<StackPanel>
<Expander Header="Section 1">
<TextBlock Text="Content for section 1." />
</Expander>
<Expander Header="Section 2">
<TextBlock Text="Content for section 2." />
</Expander>
</StackPanel>
</ScrollViewer>
실행사진
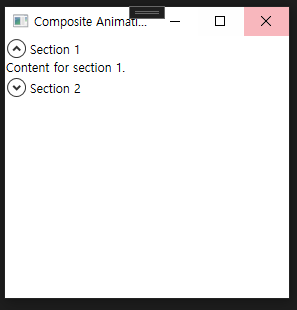
설명
- ScrollViewer: 콘텐츠가 크기를 초과할 경우 스크롤 추가.
- Expander: 클릭하여 콘텐츠를 확장/축소 가능.
8. Responsive Layout
반응형 레이아웃을 구현하려면 ViewBox 또는 Grid의 *
크기를 활용합니다.
예제 코드
<Viewbox>
<Grid>
<TextBlock Text="This is a responsive layout" FontSize="20" />
</Grid>
</Viewbox>
실행사진
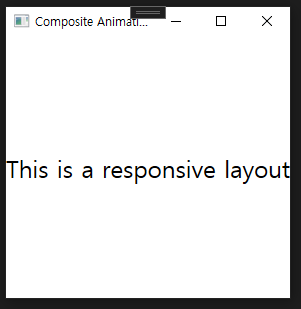
설명
- Viewbox: 요소의 크기를 부모 컨테이너에 맞게 조정.
- 반응형 디자인을 위한 간단한 방법.
요약
레이아웃 컨테이너 | 특징 | 사용 사례 |
---|---|---|
Grid | 행과 열 배치, 유연한 크기 설정 가능 | 복잡한 레이아웃 |
StackPanel | 수평/수직 정렬 | 간단한 리스트 또는 폼 |
WrapPanel | 요소가 부족한 공간에 자동 줄바꿈 | 아이콘 배치, 반응형 디자인 |
DockPanel | 요소를 가장자리에 도킹 | 헤더/사이드바 포함 레이아웃 |
Canvas | 절대 위치 배치 | 고정된 위치의 UI 요소 |
UniformGrid | 균등한 셀 배치 | 그리드 형식의 데이터 |
ScrollViewer | 스크롤 가능 | 긴 콘텐츠를 표시하는 경우 |
필요에 따라 적합한 레이아웃 컨테이너를 선택하여 프로젝트에 활용할 수 있습니다.