71
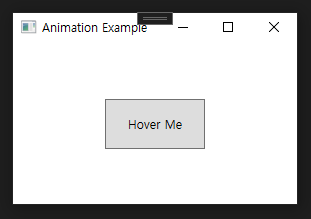
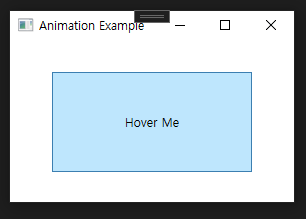
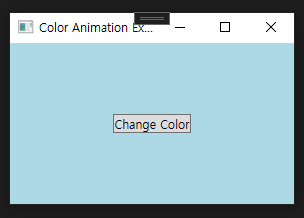
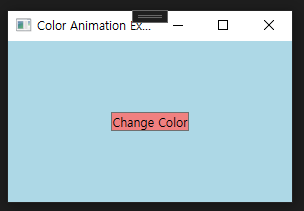
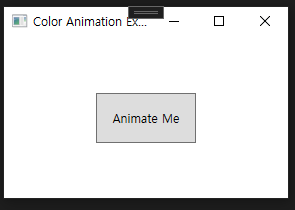
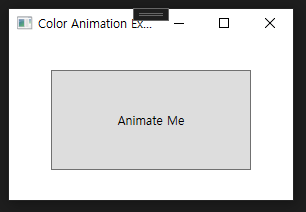
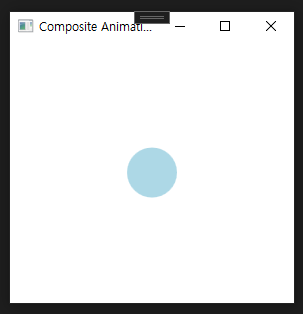
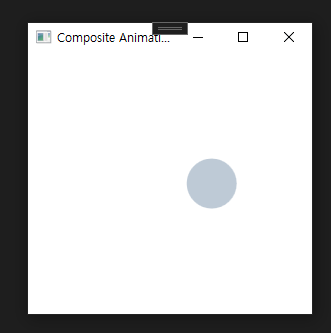
WPF의 애니메이션은 UI 요소의 속성(Property)을 시간에 따라 변경하여 동적인 사용자 경험을 제공하는 기능입니다. 애니메이션은 Storyboard와 Animation 클래스를 사용하여 구현됩니다.
주요 특징
- 속성 기반 애니메이션:
- UI 요소의 속성 값(예: 크기, 위치, 색상)을 점진적으로 변경합니다.
- Animation 클래스:
- 기본적으로 다음과 같은 애니메이션 클래스를 제공합니다.
DoubleAnimation
: 숫자 값을 점진적으로 변경.ColorAnimation
: 색상 값 변경.ThicknessAnimation
: 여백(마진) 변경.PointAnimation
: 2D 점 좌표 변경.
- 기본적으로 다음과 같은 애니메이션 클래스를 제공합니다.
- Storyboard:
- 여러 애니메이션을 그룹화하고, 시작 및 제어합니다.
- 트리거 기반 애니메이션:
- 특정 이벤트(예: 버튼 클릭, 마우스 오버)와 함께 애니메이션을 실행합니다.
WPF Animation 사용 방법
1. XAML에서 애니메이션 정의
Storyboard
를 사용하여 UI 요소에 애니메이션을 정의합니다.
2. 코드에서 애니메이션 정의
- 애니메이션 객체(
DoubleAnimation
,ColorAnimation
등)를 생성하고 속성을 설정합니다.
3. 트리거를 사용한 애니메이션 실행
- 이벤트 트리거를 사용하여 애니메이션을 자동으로 실행합니다.
예제 코드
1. 버튼 크기 애니메이션 (XAML)
<Window x:Class="WpfApp.MainWindow"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
Title="Animation Example" Height="200" Width="300">
<Grid>
<Button Content="Hover Me" Width="100" Height="50">
<Button.Triggers>
<EventTrigger RoutedEvent="Button.MouseEnter">
<BeginStoryboard>
<Storyboard>
<!-- 크기 변경 애니메이션 -->
<DoubleAnimation Storyboard.TargetProperty="Width"
From="100" To="200" Duration="0:0:0.5"/>
<DoubleAnimation Storyboard.TargetProperty="Height"
From="50" To="100" Duration="0:0:0.5"/>
</Storyboard>
</BeginStoryboard>
</EventTrigger>
<EventTrigger RoutedEvent="Button.MouseLeave">
<BeginStoryboard>
<Storyboard>
<DoubleAnimation Storyboard.TargetProperty="Width"
From="200" To="100" Duration="0:0:0.5"/>
<DoubleAnimation Storyboard.TargetProperty="Height"
From="100" To="50" Duration="0:0:0.5"/>
</Storyboard>
</BeginStoryboard>
</EventTrigger>
</Button.Triggers>
</Button>
</Grid>
</Window>
실행 결과
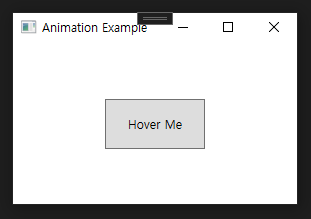
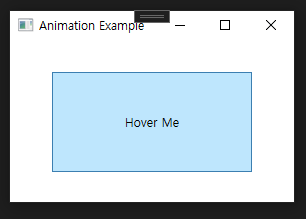
2. 배경색 변경 애니메이션 (XAML)
<Window x:Class="WpfApp.MainWindow"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
Title="Color Animation Example" Height="200" Width="300">
<Grid>
<Grid.Background>
<SolidColorBrush x:Name="BackgroundBrush" Color="LightBlue"/>
</Grid.Background>
<Button Content="Change Color" HorizontalAlignment="Center" VerticalAlignment="Center">
<Button.Triggers>
<EventTrigger RoutedEvent="Button.Click">
<BeginStoryboard>
<Storyboard>
<!-- 배경 색상 변경 애니메이션 -->
<ColorAnimation Storyboard.TargetProperty="(Grid.Background).(SolidColorBrush.Color)"
From="LightBlue" To="LightCoral" Duration="0:0:1"/>
</Storyboard>
</BeginStoryboard>
</EventTrigger>
</Button.Triggers>
</Button>
</Grid>
</Window>
실행 결과
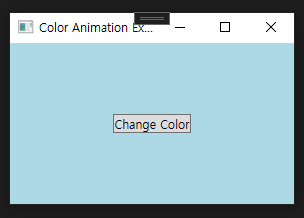
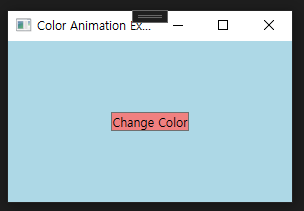
3. 코드에서 애니메이션 정의 (DoubleAnimation)
using System.Windows;
using System.Windows.Media.Animation;
namespace WpfApp
{
public partial class MainWindow : Window
{
public MainWindow()
{
InitializeComponent();
Button button = new Button
{
Content = "Animate Me",
Width = 100,
Height = 50,
HorizontalAlignment = HorizontalAlignment.Center,
VerticalAlignment = VerticalAlignment.Center
};
button.Click += Button_Click;
this.Content = button;
}
private void Button_Click(object sender, RoutedEventArgs e)
{
DoubleAnimation widthAnimation = new DoubleAnimation
{
From = 100,
To = 200,
Duration = new Duration(TimeSpan.FromSeconds(1))
};
DoubleAnimation heightAnimation = new DoubleAnimation
{
From = 50,
To = 100,
Duration = new Duration(TimeSpan.FromSeconds(1))
};
Button button = sender as Button;
// 애니메이션 실행
button.BeginAnimation(Button.WidthProperty, widthAnimation);
button.BeginAnimation(Button.HeightProperty, heightAnimation);
}
}
}
실행 결과
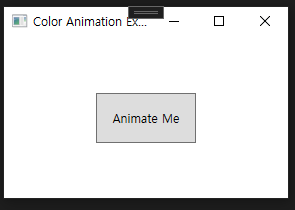
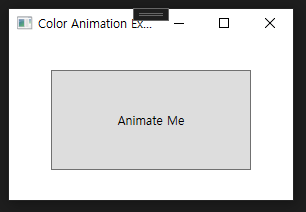
4. 복합 애니메이션 (스토리보드와 여러 속성)
<Window x:Class="WpfApp.MainWindow"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
Title="Composite Animation Example" Height="300" Width="300">
<Grid>
<Ellipse Fill="LightBlue" Width="50" Height="50">
<Ellipse.RenderTransform>
<TranslateTransform x:Name="ellipseTransform"/>
</Ellipse.RenderTransform>
<Ellipse.Triggers>
<EventTrigger RoutedEvent="Ellipse.MouseEnter">
<BeginStoryboard>
<Storyboard>
<!-- 위치 변경 -->
<DoubleAnimation Storyboard.TargetProperty="(RenderTransform).(TranslateTransform.X)"
From="0" To="200" Duration="0:0:1"/>
<!-- 색상 변경 -->
<ColorAnimation Storyboard.TargetProperty="(Fill).(SolidColorBrush.Color)"
From="LightBlue" To="LightCoral" Duration="0:0:1"/>
</Storyboard>
</BeginStoryboard>
</EventTrigger>
</Ellipse.Triggers>
</Ellipse>
</Grid>
</Window>
실행 결과
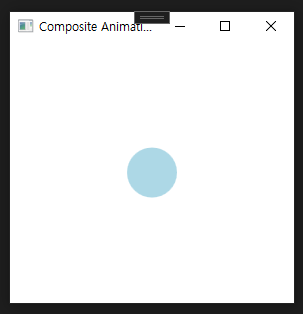
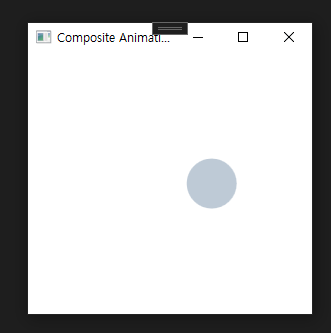
요약
- XAML 기반 애니메이션:
- 간단한 애니메이션을 정의할 때 사용.
Storyboard
와 트리거로 UI 동작 제어.
- 코드 기반 애니메이션:
- 런타임 동적 애니메이션을 구현할 때 사용.
BeginAnimation
메서드로 특정 속성에 애니메이션 적용.
- 복합 애니메이션:
- 여러 속성을 동시에 애니메이션할 때
Storyboard
를 사용.
- 여러 속성을 동시에 애니메이션할 때
WPF의 애니메이션은 UI 요소에 생동감을 주고, 사용자 경험을 풍부하게 만드는 데 매우 유용합니다.