140
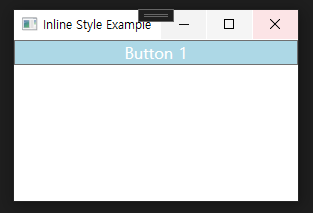
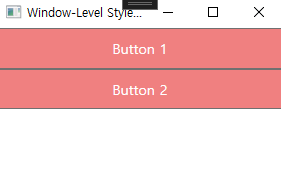
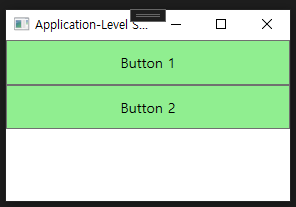
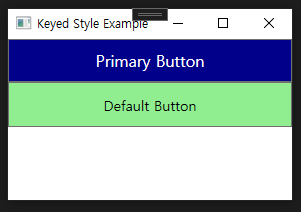
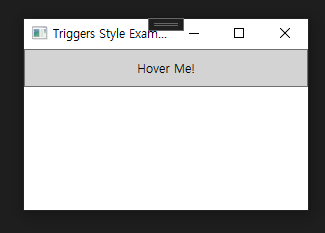
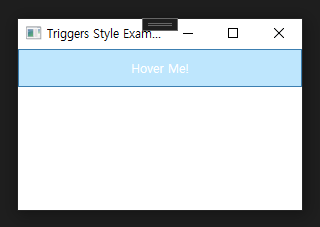
WPF의 Style은 XAML에서 UI 요소의 모양과 동작을 일관되게 정의하는 방법입니다. Style은 Setter를 통해 속성 값을 정의하고, 특정 컨트롤이나 여러 컨트롤에 적용할 수 있습니다. 이를 통해 코드를 간결하게 유지하고 디자인의 일관성을 보장할 수 있습니다.
Style의 주요 특징
- 재사용 가능: 여러 컨트롤에 동일한 스타일을 적용할 수 있습니다.
- 분리된 디자인: UI 스타일 정의를 XAML에서 분리하여 유지보수성을 높입니다.
- 동적/정적 리소스: 런타임에 스타일을 변경하거나, 정적으로 적용할 수 있습니다.
- 트리거 지원:
Style
은 조건부 스타일을 정의하기 위해 Triggers를 사용할 수 있습니다.
Style의 사용 방법
1. Inline Style
- 특정 컨트롤에 직접 Style을 정의.
2. Window-Level Style
- Window 또는 Page의 Resource에 Style 정의.
3. Application-Level Style
- App.xaml에 Style 정의하여 전체 애플리케이션에 적용.
예제 코드
1. Inline Style
<Window x:Class="WpfApp.MainWindow"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
Title="Inline Style Example" Height="200" Width="300">
<StackPanel>
<Button Content="Button 1">
<Button.Style>
<Style TargetType="Button">
<Setter Property="Background" Value="LightBlue"/>
<Setter Property="Foreground" Value="White"/>
<Setter Property="FontSize" Value="16"/>
</Style>
</Button.Style>
</Button>
</StackPanel>
</Window>
실행 결과
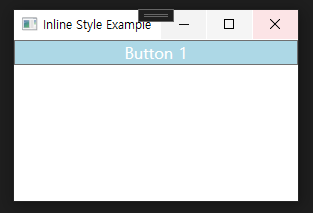
2. Window-Level Style
<Window x:Class="WpfApp.MainWindow"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
Title="Window-Level Style Example" Height="200" Width="300">
<Window.Resources>
<Style TargetType="Button">
<Setter Property="Background" Value="LightCoral"/>
<Setter Property="Foreground" Value="White"/>
<Setter Property="FontSize" Value="14"/>
<Setter Property="Padding" Value="10"/>
</Style>
</Window.Resources>
<StackPanel>
<Button Content="Button 1"/>
<Button Content="Button 2"/>
</StackPanel>
</Window>
실행 결과
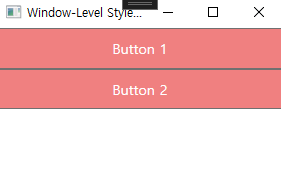
3. Application-Level Style
App.xaml
<Application x:Class="WpfApp.App"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
StartupUri="MainWindow.xaml">
<Application.Resources>
<Style TargetType="Button">
<Setter Property="Background" Value="LightGreen"/>
<Setter Property="Foreground" Value="Black"/>
<Setter Property="FontSize" Value="14"/>
<Setter Property="Padding" Value="12"/>
</Style>
</Application.Resources>
</Application>
MainWindow.xaml
<Window x:Class="WpfApp.MainWindow"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
Title="Application-Level Style Example" Height="200" Width="300">
<StackPanel>
<Button Content="Button 1"/>
<Button Content="Button 2"/>
</StackPanel>
</Window>
실행 결과
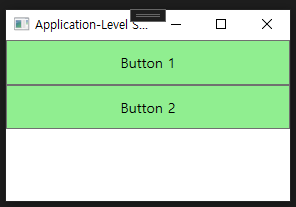
4. Keyed Style
특정 컨트롤에만 스타일을 적용하려면 키를 사용합니다.
<Window x:Class="WpfApp.MainWindow"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
Title="Keyed Style Example" Height="200" Width="300">
<Window.Resources>
<Style x:Key="PrimaryButtonStyle" TargetType="Button">
<Setter Property="Background" Value="DarkBlue"/>
<Setter Property="Foreground" Value="White"/>
<Setter Property="FontSize" Value="16"/>
<Setter Property="Padding" Value="10"/>
</Style>
</Window.Resources>
<StackPanel>
<Button Content="Primary Button" Style="{StaticResource PrimaryButtonStyle}"/>
<Button Content="Default Button"/>
</StackPanel>
</Window>
실행 결과
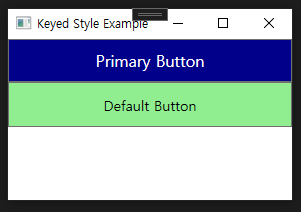
5. Triggers 포함 Style
조건부 스타일링을 위해 Triggers
를 사용합니다.
<Window x:Class="WpfApp.MainWindow"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
Title="Triggers Style Example" Height="200" Width="300">
<Window.Resources>
<Style TargetType="Button">
<Setter Property="Background" Value="LightGray"/>
<Setter Property="Foreground" Value="Black"/>
<Setter Property="Padding" Value="10"/>
<Style.Triggers>
<Trigger Property="IsMouseOver" Value="True">
<Setter Property="Background" Value="Orange"/>
<Setter Property="Foreground" Value="White"/>
</Trigger>
</Style.Triggers>
</Style>
</Window.Resources>
<StackPanel>
<Button Content="Hover Me!"/>
</StackPanel>
</Window>
실행 결과
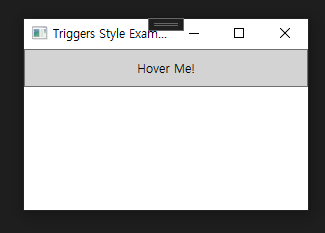
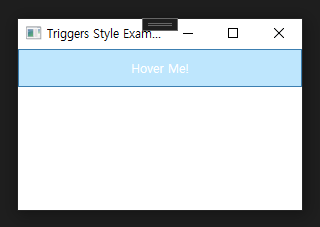
요약
- Style은 UI 요소의 모양과 동작을 통합적으로 관리하는 데 사용됩니다.
- Inline Style은 특정 컨트롤에 사용되며, Window-Level과 Application-Level Style은 여러 컨트롤에 적용됩니다.
Keyed Style
과Triggers
를 사용하면 더 정교한 스타일링이 가능합니다.- WPF 스타일은 유지보수성과 재사용성을 높이는 데 매우 유용합니다.