83
1.
1.
2.
3.
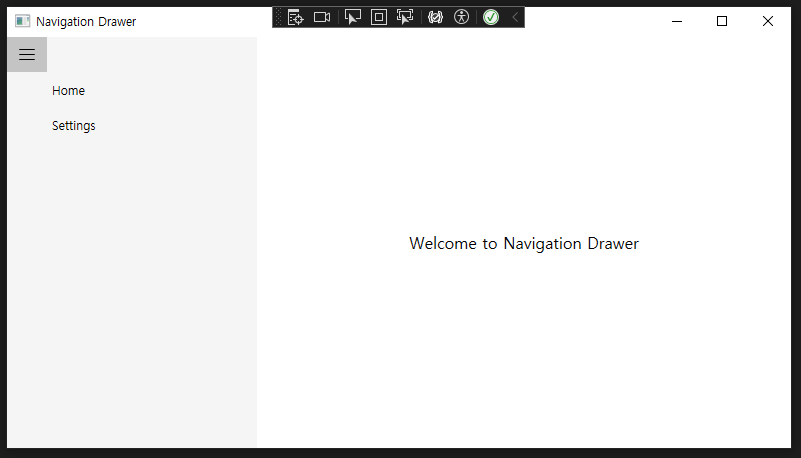
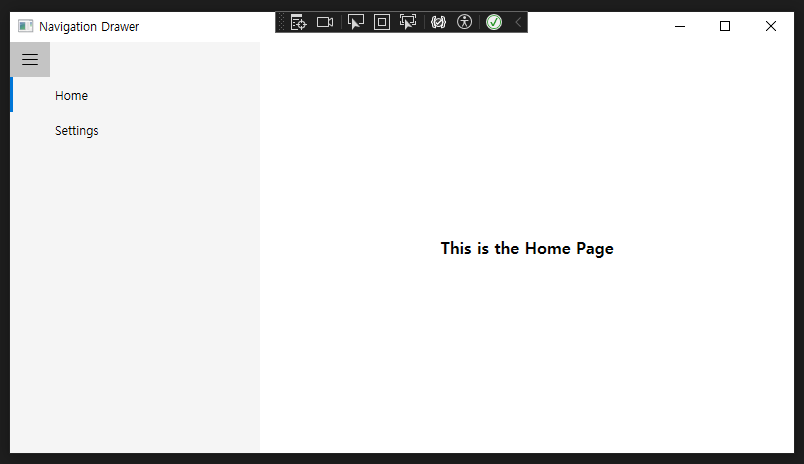
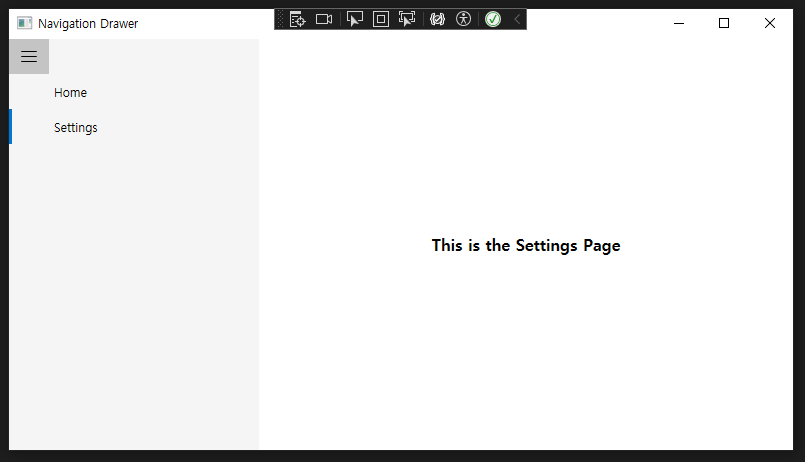
WPF 프로젝트에서 Syncfusion의 SfNavigationDrawer
컨트롤을 사용하여 **내비게이션 드로어(Navigation Drawer)**와 다른 사용자 컨트롤(UserControl) 간의 콘텐츠 전환을 구현한 예제입니다.
설정 방법
1. NuGet 패키지 설치
Syncfusion WPF 라이브러리를 설치합니다
Install-Package Syncfusion.SfNavigationDrawer.WPF
작동 원리
1. SfNavigationDrawer
- **
SfNavigationDrawer
**는 내비게이션 항목(메뉴)과 해당 콘텐츠를 분리하여 구성하는 Syncfusion의 WPF 컨트롤입니다. NavigationItem
:- 드로어 메뉴의 항목을 정의합니다. 예를 들어 “Home”과 “Settings” 항목이 있습니다.
- 각 항목에
Header
와Tag
속성을 사용하여 항목 이름과 태그(식별자)를 설정합니다.
ContentView
:- 선택된 항목에 따라 보여줄 콘텐츠를 정의하는 영역입니다. 이 영역은 기본적으로 **
Grid
**로 설정되어 있으며, 선택된 항목에 따라 해당 콘텐츠를 동적으로 변경합니다.
- 선택된 항목에 따라 보여줄 콘텐츠를 정의하는 영역입니다. 이 영역은 기본적으로 **
2. UserControl
- **
HomeControl
**과 **SettingsControl
**은 각각 “Home”과 “Settings” 메뉴에 해당하는 화면을 정의합니다. - 각각의 **
UserControl
**은 독립적인 UI 요소이며, 필요한 경우 독자적인 로직과 데이터 바인딩을 포함할 수 있습니다.
3. 이벤트 처리
SfNavigationDrawer.ItemClicked
이벤트:- 내비게이션 드로어에서 항목이 클릭될 때 발생합니다.
NavigationItemClickedEventArgs
를 통해 클릭된 항목의 정보를 확인할 수 있습니다.- 클릭된 항목의
Tag
속성에 따라 해당UserControl
을 동적으로contentViewGrid
에 추가합니다.
구현 방법
1. SfNavigationDrawerWindow.xaml
- Syncfusion의 **
SfNavigationDrawer
**를 사용하여 기본 레이아웃과 항목을 정의합니다. NavigationItem
의Tag
속성을 통해 항목을 식별합니다.ContentView
는 선택된 항목에 따라 변경될 콘텐츠를 표시하는 영역입니다.
<syncfusion:SfNavigationDrawer.Items>
<syncfusion:NavigationItem Header="Home" Tag="Home" />
<syncfusion:NavigationItem Header="Settings" Tag="Settings" />
</syncfusion:SfNavigationDrawer.Items>
NavigationDrawer_ItemClicked
이벤트는SfNavigationDrawer
의 항목 클릭 시 호출됩니다.
2. SfNavigationDrawerWindow.xaml.cs
ItemClicked
이벤트 핸들러:- 클릭된 항목의
Tag
속성을 확인하고, 해당 태그에 따라 적절한UserControl
을 생성하여 **contentViewGrid
**에 추가합니다.
- 클릭된 항목의
- 구현된 로직
private void NavigationDrawer_ItemClicked(object sender, NavigationItemClickedEventArgs e)
{
if (e.Item is NavigationItem selectedItem)
{
string tag = selectedItem.Tag?.ToString();
UserControl control = tag switch
{
"Home" => new HomeControl(), // Home UserControl
"Settings" => new SettingsControl(), // Settings UserControl
_ => null
};
if (control != null)
{
contentViewGrid.Children.Clear();
contentViewGrid.Children.Add(control);
}
}
}
- 핵심 로직
- **
e.Item
**을 통해 클릭된 항목을 가져옵니다. Tag
속성을 확인하여 클릭된 항목을 식별합니다.switch
식을 사용하여 적절한UserControl
을 생성합니다.- **
contentViewGrid.Children
**를 비우고 새로 생성된UserControl
을 추가합니다.
- **
3. HomeControl.xaml
및 SettingsControl.xaml
- 각각의 화면 콘텐츠를 정의하는 XAML 파일입니다.
UserControl
로 구현하여 재사용성을 높였습니다.- 구현된 로직
<UserControl x:Class="SynfusionWpfApp241129.HomeControl"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml">
<Grid>
<TextBlock
VerticalAlignment="Center"
HorizontalAlignment="Center"
FontSize="16"
FontWeight="Bold"
Text="This is the Home Page" />
</Grid>
</UserControl>
확장 및 활용
- 다른 항목 추가:
- 새로운
NavigationItem
과UserControl
을 추가하면 쉽게 확장할 수 있습니다. - 예: “About”, “Help” 등 추가.
- 새로운
- 데이터 바인딩:
UserControl
내부에 MVVM 패턴을 적용하여 데이터 바인딩을 사용할 수 있습니다.
- 스타일 커스터마이징:
- Syncfusion의 테마와 스타일을 적용하여 내비게이션 드로어와 항목의 UI를 사용자 지정할 수 있습니다.
- 동적 항목 로드:
SfNavigationDrawer.Items
를 코드로 동적으로 추가 및 제거할 수 있습니다.
전체 코드
SfNavigationDrawerWindow.xaml.cs
using Syncfusion.UI.Xaml.NavigationDrawer;
using System.Windows;
using System.Windows.Controls;
namespace SynfusionWpfApp
{
/// <summary>
/// SfNavigationDrawerWindow.xaml에 대한 상호 작용 논리
/// </summary>
public partial class SfNavigationDrawerWindow : Window
{
public SfNavigationDrawerWindow()
{
InitializeComponent();
}
private void NavigationDrawer_ItemClicked (object sender, NavigationItemClickedEventArgs e)
{
if (e.Item is NavigationItem selectedItem)
{
string tag = selectedItem.Tag?.ToString();
UserControl control = tag switch
{
"Home" => new HomeControl(), // 실제 Home UserControl
"Settings" => new SettingsControl(), // 실제 Settings UserControl
_ => null
};
if (control != null)
{
contentViewGrid.Children.Clear();
contentViewGrid.Children.Add(control);
}
}
}
}
}
SfNavigationDrawerWindow.xaml
<Window x:Class="SynfusionWpfApp.SfNavigationDrawerWindow"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:d="http://schemas.microsoft.com/expression/blend/2008"
xmlns:mc="http://schemas.openxmlformats.org/markup-compatibility/2006"
xmlns:syncfusion="http://schemas.syncfusion.com/wpf"
mc:Ignorable="d"
Title="Navigation Drawer" Height="450" Width="800">
<Grid>
<syncfusion:SfNavigationDrawer
x:Name="navigationDrawer"
AutoChangeDisplayMode="True"
DisplayMode="Compact"
ExpandedModeThresholdWidth="700"
ItemClicked="NavigationDrawer_ItemClicked">
<!-- Navigation Items -->
<syncfusion:SfNavigationDrawer.Items>
<syncfusion:NavigationItem Header="Home" Tag="Home" />
<syncfusion:NavigationItem Header="Settings" Tag="Settings" />
</syncfusion:SfNavigationDrawer.Items>
<!-- Content View -->
<syncfusion:SfNavigationDrawer.ContentView>
<Grid x:Name="contentViewGrid">
<TextBlock
VerticalAlignment="Center"
HorizontalAlignment="Center"
FontSize="16"
Text="Welcome to Navigation Drawer" />
</Grid>
</syncfusion:SfNavigationDrawer.ContentView>
</syncfusion:SfNavigationDrawer>
</Grid>
</Window>
HomeControl.xaml
<UserControl x:Class="SynfusionWpfApp.HomeControl"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
Height="Auto" Width="Auto">
<Grid>
<TextBlock
VerticalAlignment="Center"
HorizontalAlignment="Center"
FontSize="16"
FontWeight="Bold"
Text="This is the Home Page" />
</Grid>
</UserControl>
SettingsControl.xaml
<UserControl x:Class="SynfusionWpfApp.SettingsControl"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
Height="Auto" Width="Auto">
<Grid>
<TextBlock
VerticalAlignment="Center"
HorizontalAlignment="Center"
FontSize="16"
FontWeight="Bold"
Text="This is the Settings Page" />
</Grid>
</UserControl>
실행 사진
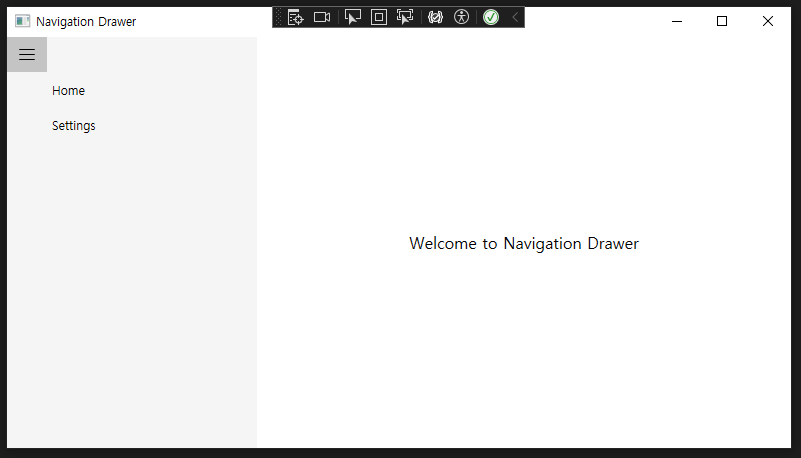
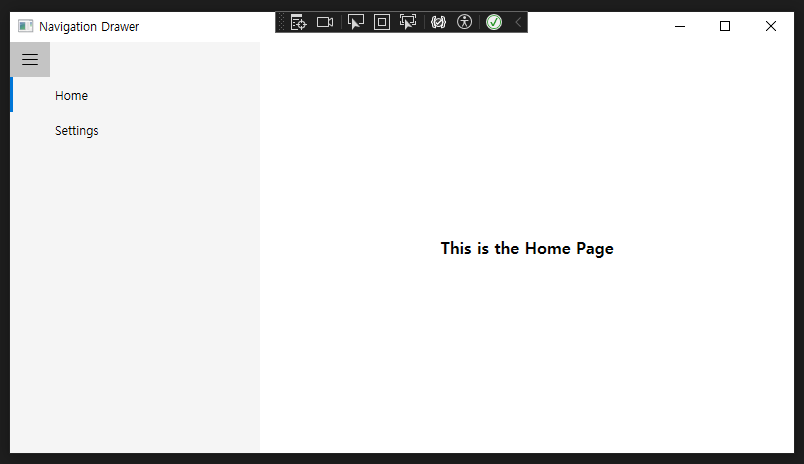
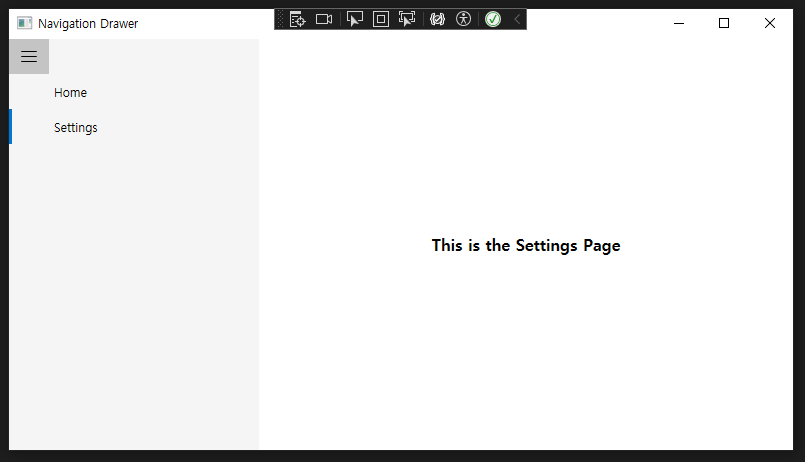