56
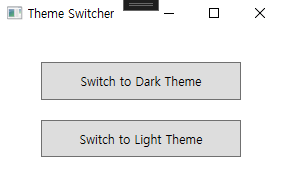
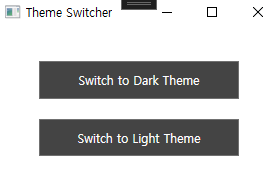
WPF의 테마는 앱 전반에서 일관된 스타일과 모양을 정의하기 위해 사용됩니다. 일반적으로 ResourceDictionary를 사용하여 XAML 스타일을 정의하고, 이를 Application 레벨에서 사용하거나 특정 컨트롤에 적용할 수 있습니다.
테마 사용 방법
- ResourceDictionary 파일 생성:
- 테마 관련 스타일 정의를 별도의 XAML 파일로 작성합니다.
- Application에서 테마 등록:
App.xaml
에서 테마 파일을 병합하여 애플리케이션 전체에 적용합니다.
- 컨트롤에 개별 적용:
- 특정 컨트롤에서 리소스를 명시적으로 참조하여 테마를 적용할 수 있습니다.
테마 비교를 위한 예시 코드
테마 정의
DarkTheme.xaml
<ResourceDictionary xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml">
<Style TargetType="Window">
<Setter Property="Background" Value="Black"/>
<Setter Property="Foreground" Value="White"/>
</Style>
<Style TargetType="Button">
<Setter Property="Background" Value="#444"/>
<Setter Property="Foreground" Value="White"/>
<Setter Property="Padding" Value="10"/>
</Style>
</ResourceDictionary>
LightTheme.xaml
<ResourceDictionary xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml">
<Style TargetType="Window">
<Setter Property="Background" Value="White"/>
<Setter Property="Foreground" Value="Black"/>
</Style>
<Style TargetType="Button">
<Setter Property="Background" Value="#DDD"/>
<Setter Property="Foreground" Value="Black"/>
<Setter Property="Padding" Value="10"/>
</Style>
</ResourceDictionary>
App.xaml에서 테마 적용
<Application x:Class="WpfApp.App"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
StartupUri="MainWindow.xaml">
<Application.Resources>
<ResourceDictionary>
<ResourceDictionary.MergedDictionaries>
<!-- Dark Theme 적용 -->
<ResourceDictionary Source="Themes/DarkTheme.xaml"/>
</ResourceDictionary.MergedDictionaries>
</ResourceDictionary>
</Application.Resources>
</Application>
MainWindow.xaml
<Window x:Class="WpfApp.MainWindow"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
Title="Theme Switcher" Height="200" Width="300">
<StackPanel HorizontalAlignment="Center" VerticalAlignment="Center">
<Button Content="Switch to Dark Theme" Width="200" Margin="10" Click="SwitchToDarkTheme_Click"/>
<Button Content="Switch to Light Theme" Width="200" Margin="10" Click="SwitchToLightTheme_Click"/>
</StackPanel>
</Window>
테마 동적으로 변경
테마를 동적으로 변경하려면 ResourceDictionary
를 코드에서 교체해야 합니다.
App.xaml.cs
using System;
using System.Windows;
namespace WpfApp
{
public partial class App : Application
{
public void ChangeTheme(string theme)
{
// 새로운 테마 ResourceDictionary 로드
var newTheme = new ResourceDictionary
{
Source = new Uri($"Themes/{theme}Theme.xaml", UriKind.Relative)
};
// 기존 리소스 딕셔너리 교체
Resources.MergedDictionaries.Clear();
Resources.MergedDictionaries.Add(newTheme);
}
}
}
MainWindow.xaml.cs
using System.Windows;
namespace WpfApp
{
public partial class App : Application
{
public void ChangeTheme (string theme)
{
var dict = new ResourceDictionary();
switch (theme)
{
case "Dark":
dict.Source = new System.Uri("DarkTheme.xaml", System.UriKind.Relative);
break;
case "Light":
dict.Source = new System.Uri("LightTheme.xaml", System.UriKind.Relative);
break;
}
// 기존 리소스 제거 및 새로운 테마 추가
this.Resources.MergedDictionaries.Clear();
this.Resources.MergedDictionaries.Add(dict);
}
}
}
실행 결과
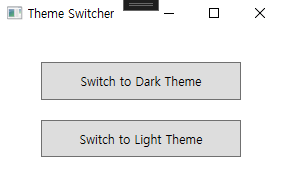
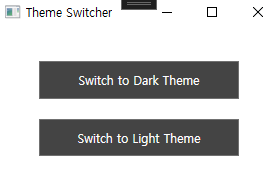
요약
- WPF 테마는
ResourceDictionary
를 통해 관리되며, 전체 애플리케이션에 일관된 스타일을 적용하거나 개별 컨트롤에 적용할 수 있습니다. - 동적으로 테마를 변경하려면
Application.Resources.MergedDictionaries
를 활용합니다.