75
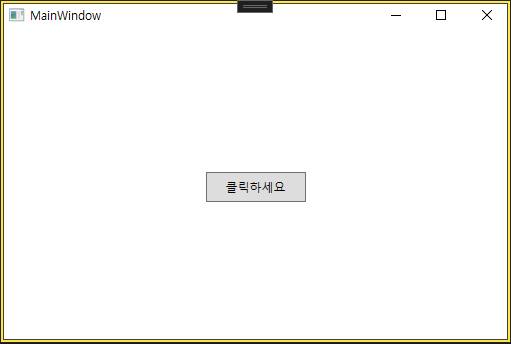
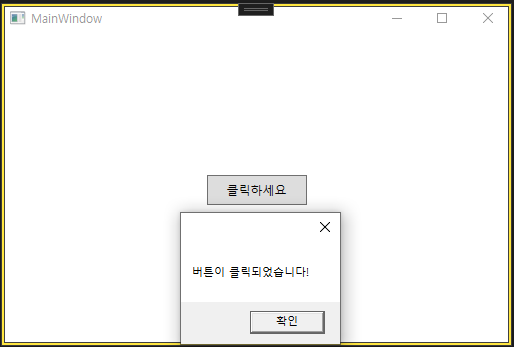
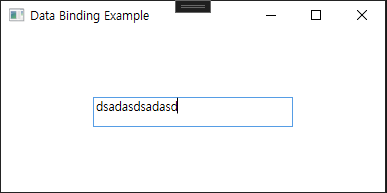
XAML(Extensible Application Markup Language)은 Microsoft에서 개발한 선언적 XML 기반 언어로, WPF(Windows Presentation Foundation), UWP(Universal Windows Platform), Xamarin.Forms 등에서 사용자 인터페이스(UI)를 정의하는 데 사용됩니다. XAML은 프로그램의 UI 요소를 선언적으로 표현할 수 있어 코드로 UI를 구성하는 것보다 직관적이고 가독성이 높습니다. XAML은 UI 요소들을 계층 구조로 정의하며, 각 요소의 속성과 자식 요소도 설정할 수 있습니다.
XAML의 기본 구조
<Window x:Class="WpfApp.MainWindow"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
Title="MainWindow" Height="350" Width="525">
<Grid>
<Button Content="클릭하세요" Width="100" Height="30" VerticalAlignment="Center" HorizontalAlignment="Center"/>
</Grid>
</Window>
실행 결과
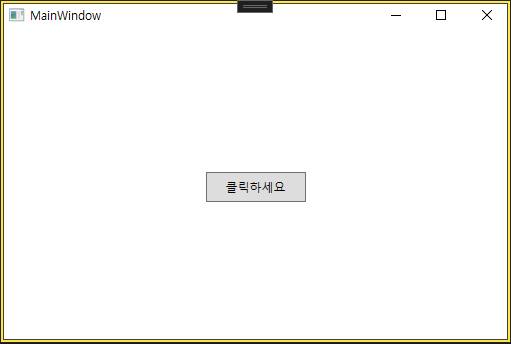
설명:
<Window>
: WPF 애플리케이션의 메인 윈도우를 나타냅니다.xmlns
속성: 기본 XAML 네임스페이스와x:
접두사를 사용하는 XAML 네임스페이스를 정의합니다.<Grid>
: 자식 요소를 배치하기 위한 컨테이너입니다.<Button>
: UI에 표시될 버튼을 정의하며,Content
속성을 사용해 버튼 텍스트를 지정합니다.
XAML에서 속성과 이벤트
XAML은 속성 설정과 이벤트 처리도 가능합니다. 예를 들어 버튼 클릭 이벤트를 처리하는 코드와 연결할 수도 있습니다.
XAML 코드
<Window x:Class="WpfApp.MainWindow"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
Title="MainWindow" Height="350" Width="525">
<Grid>
<Button Content="클릭하세요" Click="Button_Click" Width="100" Height="30" VerticalAlignment="Center" HorizontalAlignment="Center"/>
</Grid>
</Window>
C# 코드 (Code-behind)
using System.Windows;
namespace WpfApp
{
public partial class MainWindow : Window
{
public MainWindow()
{
InitializeComponent();
}
private void Button_Click(object sender, RoutedEventArgs e)
{
MessageBox.Show("버튼이 클릭되었습니다!");
}
}
}
실행 결과
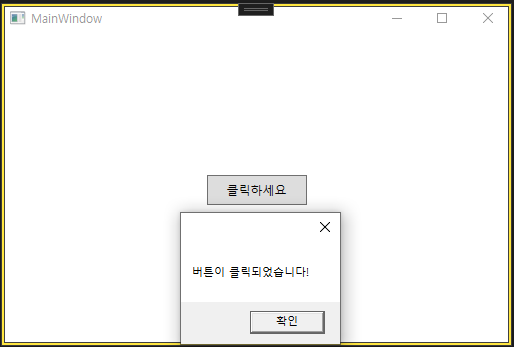
데이터 바인딩 예시
XAML은 데이터 바인딩 기능을 제공하여 UI 요소가 데이터와 동기화될 수 있도록 합니다.
XAML 코드
<Window x:Class="WpfApp.MainWindow"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
Title="Data Binding Example" Height="200" Width="400">
<Grid>
<TextBox Text="{Binding Name, UpdateSourceTrigger=PropertyChanged}" Width="200" Height="30" VerticalAlignment="Center" HorizontalAlignment="Center"/>
</Grid>
</Window>
C# 코드
using System.ComponentModel;
using System.Runtime.CompilerServices;
using System.Windows;
namespace WpfApp
{
public partial class MainWindow : Window, INotifyPropertyChanged
{
private string _name;
public string Name
{
get => _name;
set
{
_name = value;
OnPropertyChanged();
}
}
public MainWindow()
{
InitializeComponent();
DataContext = this;
}
public event PropertyChangedEventHandler PropertyChanged;
protected void OnPropertyChanged([CallerMemberName] string propertyName = null)
{
PropertyChanged?.Invoke(this, new PropertyChangedEventArgs(propertyName));
}
}
}
실행 결과
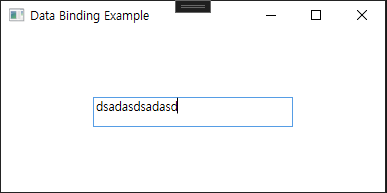
설명:
{Binding Name}
: XAML의TextBox
가 C#의Name
속성과 바인딩됩니다.DataContext
: XAML UI 요소의 기본 데이터 컨텍스트를 설정하여 데이터 바인딩이 가능합니다.