35
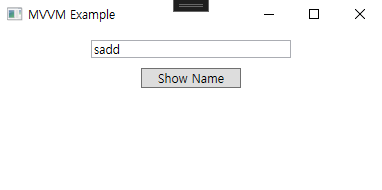
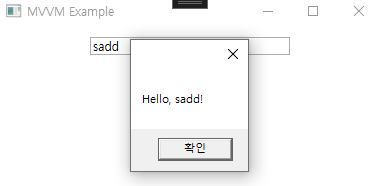
WPF에서 명령은 사용자 인터페이스(UI)에서 발생하는 액션을 처리하는 메커니즘을 제공합니다. 명령을 사용하면 버튼 클릭이나 메뉴 선택 등의 사용자 인터페이스 동작을 뷰모델이나 코드 비하인드에 연결할 수 있어, UI 로직과 비즈니스 로직의 분리를 가능하게 합니다.
WPF 명령은 기본적으로 ICommand
인터페이스를 구현하며, Execute()
메서드와 CanExecute()
메서드로 구성됩니다.
명령과 메서드의 차이점
- 명령은 UI에서 발생한 이벤트를 처리할 때 사용되며, 사용자의 액션(예: 버튼 클릭)을 뷰모델의 메서드에 연결할 수 있습니다.
- 메서드는 특정 로직을 수행하는 일반적인 C# 코드로, 명령과는 달리 UI 이벤트와 직접적인 연결이 없습니다.
명령의 구성 요소
ICommand
인터페이스: WPF의 명령 시스템은ICommand
인터페이스로 정의되며,Execute()
와CanExecute()
메서드를 제공합니다.RelayCommand
클래스: 명령을 쉽게 구현할 수 있도록 돕는 클래스입니다.
활용 예시
사용자가 버튼을 클릭하면 메시지를 표시하는 명령을 구현해 보겠습니다.
RelayCommand.cs
using System;
using System.Windows.Input;
namespace WpfApp
{
public class RelayCommand : ICommand
{
private readonly Action _execute;
private readonly Func<bool> _canExecute;
public event EventHandler CanExecuteChanged;
public RelayCommand(Action execute, Func<bool> canExecute = null)
{
_execute = execute ?? throw new ArgumentNullException(nameof(execute));
_canExecute = canExecute;
}
public bool CanExecute(object parameter)
{
return _canExecute == null || _canExecute();
}
public void Execute(object parameter)
{
_execute();
}
public void RaiseCanExecuteChanged()
{
CanExecuteChanged?.Invoke(this, EventArgs.Empty);
}
}
}
ViewModel에서 명령 사용 예시
using System.ComponentModel;
using System.Runtime.CompilerServices;
using System.Windows;
using System.Windows.Input;
namespace WpfApp
{
public class MainViewModel : INotifyPropertyChanged
{
private string _name;
public string Name
{
get => _name;
set
{
_name = value;
OnPropertyChanged();
}
}
public ICommand ShowNameCommand { get; }
public MainViewModel()
{
ShowNameCommand = new RelayCommand(ShowName);
}
private void ShowName()
{
MessageBox.Show($"Hello, {Name}!");
}
public event PropertyChangedEventHandler PropertyChanged;
protected void OnPropertyChanged([CallerMemberName] string propertyName = null)
{
PropertyChanged?.Invoke(this, new PropertyChangedEventArgs(propertyName));
}
}
}
View (XAML)
<Window x:Class="WpfApp.MainWindow"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
Title="MVVM Example" Height="200" Width="400">
<Grid>
<StackPanel Margin="10">
<TextBox Text="{Binding Name, UpdateSourceTrigger=PropertyChanged}" Width="200" Margin="0,0,0,10"/>
<Button Content="Show Name" Command="{Binding ShowNameCommand}" Width="100"/>
</StackPanel>
</Grid>
</Window>
MainWindow.xaml.cs
using System.Windows;
namespace WpfApp
{
public partial class MainWindow : Window
{
public MainWindow()
{
InitializeComponent();
DataContext = new MainViewModel();
}
}
}
실행 결과
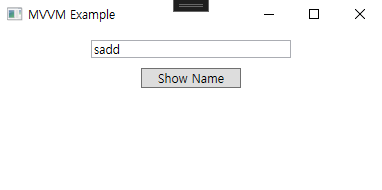
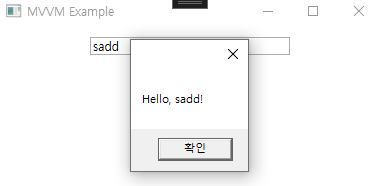
코드 설명
- MainWindow.xaml은 View로서 UI 요소를 구성하고,
DataContext
에 바인딩된 ViewModel과 상호작용합니다. - MainViewModel.cs는
Name
속성과ShowNameCommand
명령을 포함하여 사용자 입력과 명령 실행을 처리합니다. - RelayCommand.cs는 명령을 구현하기 위한 유틸리티 클래스입니다.
명령 사용의 장점
- UI 로직과 비즈니스 로직 분리: 명령은 UI와 비즈니스 로직의 의존성을 줄이고 코드의 유지 보수성을 높입니다.
- 재사용성: 명령 객체는 여러 UI 요소에서 재사용할 수 있습니다.
- 조건부 실행:
CanExecute
메서드를 통해 명령 실행 가능 여부를 조건부로 지정할 수 있습니다.
이 예제를 통해 WPF의 명령이 어떻게 사용되고 ViewModel과 연결되는지를 이해할 수 있습니다.